In order to retrive the correct informations about the user location on a mobile device, the application uses the LocationManager class located in the android.location package.
This package is an API that is part of Google Play Services and provides a more powerful, high-level framework that automatically handles location providers.
Using the LocationManager class, the application can obtain periodic updates of the device’s geographical locations (Longitude, Latitude, Altitude).
To get user location in Android is done using callback method.
The application has to indicate that it would like receive location updates from the “Location Manager” object by calling the requestLocationUpdates() and passing it a Location Listener, that implement a set of usefull callback that the Location Manager call when the user location changes or when the status of the service changes.
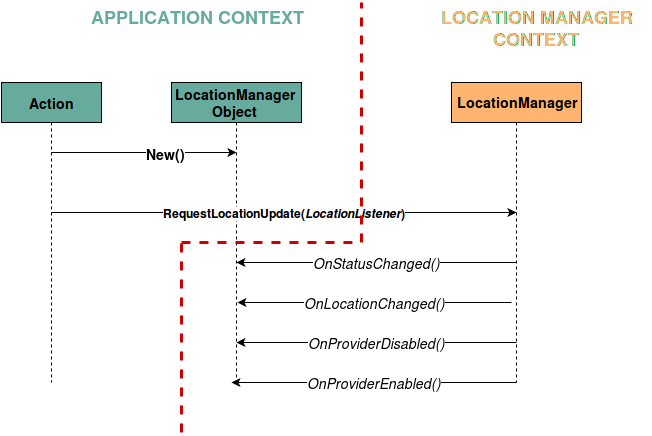
Implementation
The GpsClass.java file is built in two principal steps:
- get a reference to the Location Manager class using the getSystemService() method;
- register the application for a request for changes in locations using the requestLocationUpdates() method;
The first step is done getting the handle to a system-level service related to the location:
locationManager = (LocationManager) mContext.getSystemService(LOCATION_SERVICE);
this handle is used for controlling location updates.
The second step is done via the requestLocationUpdates():
locationManager.requestLocationUpdates(LocationManager.NETWORK_PROVIDER, MIN_TIME_BW_UPDATES, MIN_DISTANCE_CHANGE_FOR_UPDATES, this)
This method takes four parameters:
provider: The name of the provider with which you register. In this case the provider requested is the “NETWORK_PROVIDER” (it is possible use also the GPS_PROVIDER), This provider determines location based on availability of cell tower and WiFi access points. Results are retrieved by means of a network lookup.
minTime: The minimum time interval for notifications, in milliseconds.
private static final long MIN_TIME_BW_UPDATES = 1000 * 60 * 1; //1 minute
minDistance: The minimum distance interval for notifications, in meters.
private static final long MIN_DISTANCE_CHANGE_FOR_UPDATES = 10; // 10 meters
listener: An object whose onLocationChanged() method will be called for each location update. In this case the same class that call the method (for this reason the class implement the LocationListener class)
public class GpsClass extends Service implements LocationListener {
Each 10 meters or every 1 minute, the LocationManager is called and and calculates the GPS coordinates with the getLastKnownLocation method of the locationManager class.
location = locationManager.getLastKnownLocation(LocationManager.NETWORK_PROVIDER);
This method return the exact location of the device: latitude, longitude and altitude providing by the NETWORK_PROVIDER.
Before to get the geographical location it is necessary verify the network and the GPS status:
// Getting GPS status
isGPSEnabled = locationManager.isProviderEnabled(LocationManager.GPS_PROVIDER);// Getting network status
isNetworkEnabled = locationManager.isProviderEnabled(LocationManager.NETWORK_PROVIDER);
if both are not available, is not possible calculate the location and it is shown a Dialog for the activation of the GPS/WIFI sensors.
Methods of the class
The class provides the following methods:
public double calculateDistance(Location locationA, Location locationB){
Used to calculate the distance between two different location passed in input.
This method uses the function distanceTo belonging to the android.location.Location class.
it returns the approximate distance in meters between this location and the given location.
public void stopUsingGps(){
Used to stop the GPS updates;
public double getLatitude(){
Used to get the latitude value contained in the global variable latitude;
public double getLongitude(){
Used to get the longitude value contained in the global variable longitude;
public double getAltitude(){
Used to get the altitude value contained in the global variable altitude;
Manifest file
Finally to use GPS functionality in the android application, is necessary add the ACCESS_FINE_LOCATION permission to the AndroidManifest.xml
<uses-permission android:name=”android.permission.ACCESS_FINE_LOCATION” />
this permission allow the appllication to access precise location.